In place editing
In place editing dynamically shows an entry form containing data, typically in response to a user click. The user can enter data and close the form.
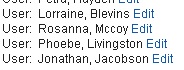
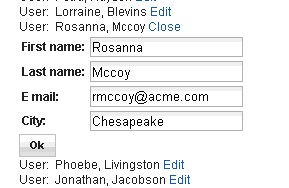
How this works
AninPlaceForm
control
contains the controls used in the form.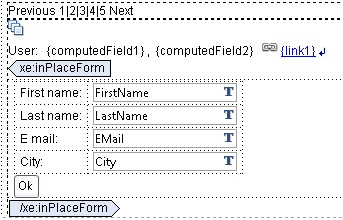
inPlaceForm
control content can be
displayed and hidden by calling the following server-side methods:var c = getComponent("inPlaceForm1") // inPlaceForm1 being the ID of the control
c.show()
c.hide()
c.toggle()
The control automatically creates and inserts the child controls into the JSF tree, or deletes them, as necessary. The control can be located with a repeat and maintains a list of contexts in which it is visible. The controls are removed from the JSF tree when there is no longer valid contexts.
The show()
and toggle()
methods
have an optional map to emulate URL parameters. The content of the
map is temporarily pushed to the requestScope
and
removed after the request is processed.
The content of an inPlaceForm
control
is not loaded unless the form is used, minimizing the runtime cost.
Implementing an OK
button in the
form
Implementing an OK
button is not as trivial
as calling show()
or hide()
. Some
server-side processing should be done, for example, saving the document.
You should not redirect to a different page if the update is successful.
You should stay on the same page and refresh the part of the page
showing the new values of the edited data.- Add a simple button not a submit button.
- Add a server-side event that saves the document then hides the
form content. This can be done with two simple actions:
Save Document
andExecute Script
.IfSave Document
succeeds,Execute Script
runs. It should simply hide the form:var c = getComponent("inPlaceForm1") c.hide()
Have the
onclick
event do a partial refresh of the edited value. The refresh ID should contain the entire form plus the edited value.If the form is editing a row within the repeat control, refresh the entire repeat control:
Accessing the current context
TheinPlaceForm
control,
as well as its children, can access the current page context. This
means, for example, that an inPlaceForm
control located
in a repeat can access the current repeat context. Here is an example
of a form opening a document and using a document data source for
the current note ID:<xe:inPlaceForm id="inPlaceForm1">
<xp:panel>
<xp:this.data>
<xp:dominoDocument
var="document1" formName="Contact" action="editDocument"
documentId="#{ javascript:row.getNoteID() }"
ignoreRequestParams="true" >
</xp:dominoDocument>
</xp:this.data>
To force the data source to use
the computed properties and prevent it from using the actual requests
parameters, specify ignoreRequestParams="true"
.