Dynamic content
The Dynamic Content control contains alternate sets of content that can be presented as desired.
Here is an example where the control can either display a view or a form when the user clicks a link. The browser in fact stays on the same page as the click triggers a partial refresh of the content of the control. On the server side, the dynamic content control is replacing its children.
All Contacts View
: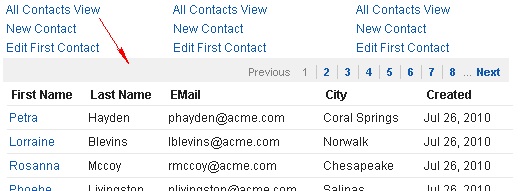
Edit First Contact
: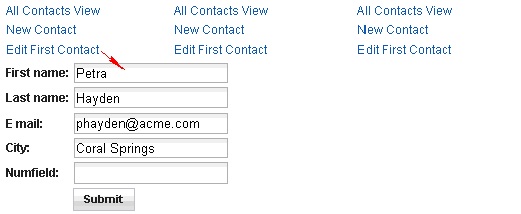
The Dynamic Content control
The Dynamic Content control contains any number of facets. Each facet represents one UI that can be displayed. Use thekey
property to identify
a facet for reference.<xe:dynamicContent id="dynp" defaultFacet="view" useHash="true" >
<xp:this.facets>
<xp:panel xp:key="view" id="panel1" >
<xp:viewPanel rows="10" id="viewPanel1" var="row" >
...
</xp:viewPanel>
</xp:panel>
<xp:panel xp:key="contact" id="panel2" >
<xp:this.data>
<xp:dominoDocument var="document1" formName="Contact" >
</xp:dominoDocument>
</xp:this.data>
...
</xp:panel>
</xp:this.facets>
</xe:dynamicContent>
dynp
is the identity
of the Dynamic Content control and view
is the identity
of a facet.var c = getComponent("dynp")
c.show("view")
show()
method
has an optional parameter used to pass URL parameters to the control
children. Those parameters are added temporarily to the requestParameterMap
and
can be consumed by the controls and complex types. Built-in data sources,
like the document data source, are reading the parameters from this
map.var c = getComponent("dynp")
c.show("contact",{action:'editDocument'
,documentId:dataAccess.firstContactID})
A typical
link implementation calls the show()
method and partially
refreshes the Dynamic Content control.
partialExecute false
Default content
Initially a Dynamic Content control can display nothing or can display one of the facets. Specify thedefaultFacet
property for initial content.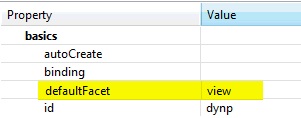
Keeping track of the content
It is sometimes interesting to keep track of the current facet being displayed, as well as the parameters, in the browser URL. This allows a user to bookmark the page and to use the back button. Unfortunately changes to the URL force the browser to refresh and send a request to the server. The only URL update that doesn't trigger a page refresh is when the#
part of the URL is modified. The technique
of using this #
part is well known in JavaScript.
The Dynamic Content control can automatically manage this if the following
property is set:useHash true
http://.../XPagesExt.nsf/Core_DynamicPage.xsp#content=contact&action=editDocument&documentId=8FA
A Dojo listener is also installed for tracking the URL changes by the user (going to a bookmark or using the back or next button). When a change is detected, an Ajax request is automatically sent to the server and the content is updated.
XSP.showContent("#{id:dynp}","view")
#content=view
On
the server side, the parameters from the hash are automatically added
to the requestMap
or the requestParameterMap
,
making it transparent to the data sources and other controls.
Implementing the OK button
Do not use a regular submit button as this would redirect to a different page on success. Instead, the button should be a simple button with the following simple actions: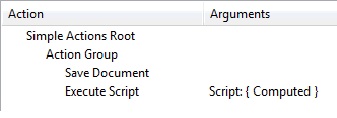
var c = getComponent("dynp")
c.show("view")

Clicking on a view column
When a click happens on a view column, you would like to dynamically switch to the entry form without refreshing the entire page. A solution consists in adding anonclick
event to the view column with one of the
techniques to change the dynamic content (server-side code, client-side
code, or relative URL). Here is an example using server-side code:var c = getComponent("dynp")
var id = row.getNoteID()
c.show("contact",{action:'editDocument',documentId:id})