In this lesson, you customize the product object to define
the user data and add the corresponding set/get methods to access
the custom data.
About this task
The class Product.java is to define
the Plain Old Java Object (POJO) for the Product noun. The data model
POJO has a flat structure. It contains strings such as name, partNumber,
short description, and more. It also creates the set/get methods so
that to access the corresponding attribute data from the Product noun.
Since warranty is the userData against Product, you need to customize
the POJO to define the user data and add the corresponding set/get
methods to access the custom data.
Procedure
- Launch your Eclipse for developing the Android application.
- Ensure that you have the Android 2.2 package installed.
- Click .
- Scroll to the Android 2.2 (API 8) package.
- If the status is Not installed, click
the package and click Install 6 packages.
When the status changes to
Installed, continue
with the next step.
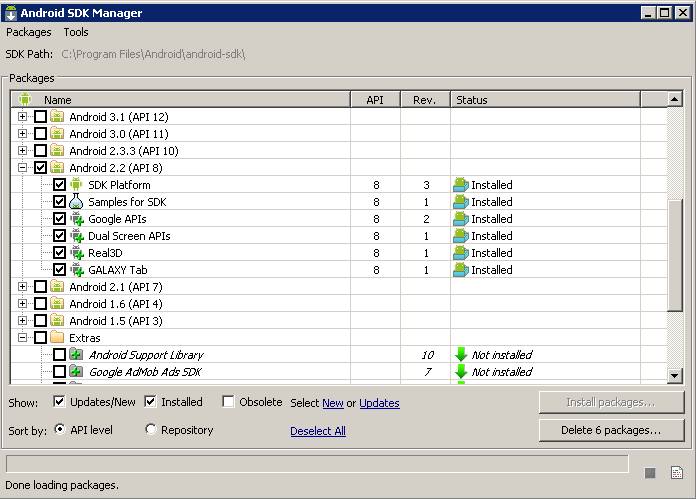
- Create an Android project and import the Android sample
code.
Note: Depending on your version of Eclipse, you can
have different ways of importing sample code.
- Click
- Enter the Project name to be MyProject,
choose to create project from existing source
- Browse the root location to be WCDE_installdir\components\store-enhancements\samples\Android\Madisons-AndroidNativeMobile
Note: Within the WCDE_installdir\components\store-enhancements\samples\Android folder, extract the Madisons-AndroidNativeMobile.zip compressed
file first.
- Select the option Google APIs with platform
2.2. Click Finish to create the project.
- In the Package Explorer view of
the Eclipse, navigate to . Open the file Product.java.
- Scroll to the bottom of the existing field definitions
private String parentCatEntryId;
private boolean isGiftItemInCart;
private boolean isSubscriptionItem;
- Add two new fields to the class as shown. The first field
stores the warrantyTerm while the second field
stores the warrantyType.
/**
* Define the private string for the user data warrantyTerm
*/
private String warrantyTerm;
/**
* Define the private string for the user data warrantyType
*/
private String warrantyType;
- Add getter and setter methods for the new fields so that
the user data is accessible.
public String getWarrantyTerm() {
return warrantyTerm;
}
public void setWarrantyTerm(String warrantyTerm) {
this.warrantyTerm = warrantyTerm;
}
public String getWarrantyType() {
return warrantyType;
}
public void setWarrantyType(String warrantyType) {
this.warrantyType = warrantyType;
}
- Save and close the file.
Note: You also can
add the getter and setter methods for the new fields by right-clicking
and selecting Source > Generate Getters and Setters from
the context menu.