WebSphere Commerce Search interactions
Search server architecture
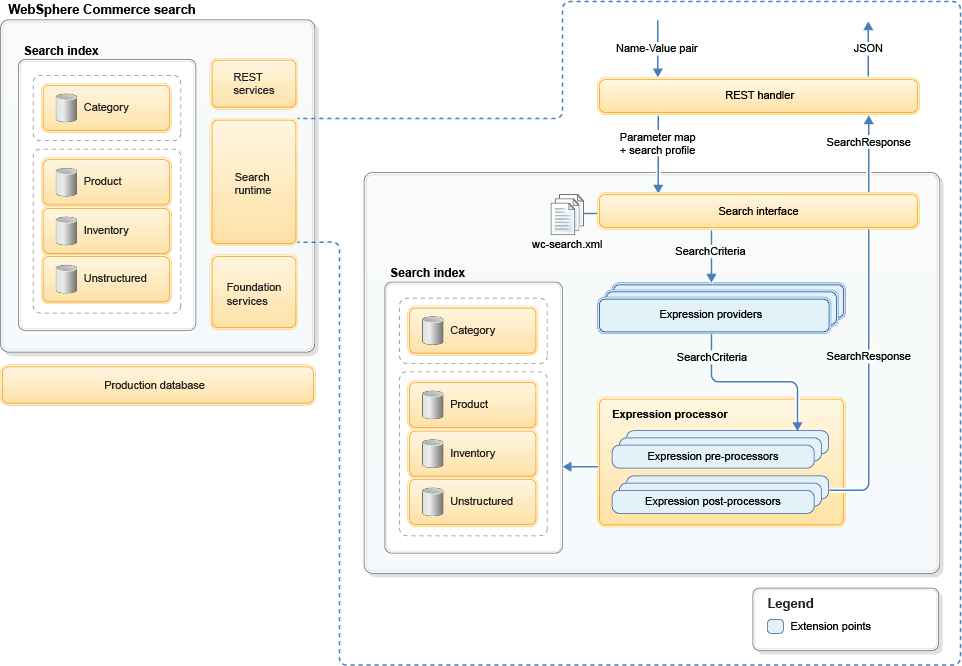
- The REST service mapper is an Apache Wink application that uses configurations to map a REST URL to a resource handler. This handler then calls the embedded search run time.
- The search run time is a pluggable programming framework that allows search expressions to be run by using properties that are defined in a configuration-based search profile. Once a search expression is composed, the runtime then forwards the request to the embedded Solr run time for processing.
- The foundation service is the WebSphere Commerce core run time that can provide basic server services, such as the JDBC Query Service to access the WebSphere Commerce database.
Example
<wcf:rest var="catalogNavigationView1" url="${searchHostNamePath}$searchContextPath}/store/${WCParam.storeId}/productview/${restType}">
<Resource name="productview"
<GetUri uri="store/{storeId}/productview/{partNumber}"
description="Get product by part number"
searchProfile="IBM_findProductByPartNumber_Details,IBM_findProductByPartNumber_Summary"/>
The Apache wink runtime then turn it into a REST request. A Java resource handler is used to handle the processing.
@Path("store/{storeId}/productview")
@Description("This class provides RESTful services to get the ProductView details.")
@Encoded
public class ProductViewHandler extends AbstractSearchResourceHandler {
store/{storeId}/productview/{partNumber}
URL is mapped to the
following method in bold:
@GET
@Path(BY_PART_NUMBER)
@Produces({ APPLICATION_JSON, APPLICATION_XML, APPLICATION_XHTML_XML,
APPLICATION_ATOM_XML})
@Description("Gets products by part number.")
@AdditionalParameterList({
@ParameterDescription(description = PARAMETER_ASSOCIATION_TYPE_DESCRIPTION, required = false, name = PARAMETER_ASSOCIATION_TYPE, multipleValue = true),
@ParameterDescription(description = PARAMETER_CATALOG_ID_DESCRIPTION, required = false, name = PARAMETER_CATALOG_ID),
@ParameterDescription(description = PARAMETER_CURRENCY_DESCRIPTION, required = false, name = PARAMETER_CURRENCY, valueProviderClass = CurrencyIdProvider.class),
@ParameterDescription(description = PARAMETER_ENTITLEMENT_CHECK_DESCRIPTION, typeClass=Boolean.class, required = false, name = PARAMETER_CHECK_ENTITLEMENT),
@ParameterDescription(description = PARAMETER_ATTACHMENT_FILTER_DESCRIPTION, required = false, name = PARAMETER_ATTACHEMENT_FILTER) })
@ResponseSchema(valueSamplePath = "ProductViewHandler/productdetails.json", responseCodes = {
@ResponseCode(code = 200, reason = RESPONSE_200_DESCRIPTION),
@ResponseCode(code = 400, reason = RESPONSE_400_DESCRIPTION),
@ResponseCode(code = 401, reason = RESPONSE_401_DESCRIPTION),
@ResponseCode(code = 403, reason = RESPONSE_403_DESCRIPTION),
@ResponseCode(code = 404, reason = RESPONSE_404_DESCRIPTION),
@ResponseCode(code = 500, reason = RESPONSE_500_DESCRIPTION) })
public Response findProductByPartNumber(
@PathParam(PARAMETER_STORE_ID) @ParameterDescription(description = PARAMETER_STORE_ID_DESCRIPTION,
valueProviderClass = StoreIdProvider.class, required = true) String storeId,
@PathParam(PART_NUMBER) @ParameterDescription(description = "The product part number.") String partNumber) {
The handler then calls the embedded search runtime code, which calls the wc-search.xml file by using the search profile names to look up the provider and preprocessors to call. This method is similar to a factory assembly line, where each business component can contribute its own piece of the search expression into the main query. After the search run time constructs the final Solr query, it calls Solr and processes the output by using postprocessors that are defined in the search profile. Then, the REST handler outputs the response.
The search expression is represented by the SearchCriteria Object, which contains a set of control parameters in the form of name-value parameter maps.
SolrSearchEDi > com.ibm.commerce.foundation.internal.server.services.search.query.solr.SolrSearchEDismaxQueryPreProcessor
invoke(SelectionCriteria, Object) ENTRY Search profile: 'IBM_findProductsBySearchTerm', Control parameters:
'_wcf.search.exclude.type=[],' 'DynamicKitReturnPrice=[true],' '_wcf.search.currency=[USD],' '_wcf.search.manufacturer=[],
' '_wcf.search.runAsId=[-1002],' '_wcf.search.contract=[10001],' '_wcf.search.catalog=[10052],''_wcf.search.catalog=[10052],
''_wcf.search.catalog=[10052],' '_wcf.search.internal.response.format=[json],'
After a search expression is composed, the run time forwards the request to the embedded Solr run time for processing.
If there are any parameters that you want to pass in from the storefront, but do not want to specify them in the findProductByPartNumber method for example, you can add it to the control parameter mapper in the wc-component.xml file. This file maps from the name-value pair into the control parameter in the SearchCriteria object.
Search programming model
The search runtime framework is driven by a programming pattern that is similar to a factory assembly line, where each business component can contribute its own piece of the search expression into the main query, which is then run against the Solr search engine.

- Search Expression Provider
- Depending on the nature of the request, that is, the search profile, other business components might get involved, such as Marketing for search-based merchandising rules, or contracts for entitlement. Each business component is responsible for contributing a portion of the search expression, which gets combined with the main search expression generated by the Search REST services.
- Search Expression Processor
- A search processor is the central processing unit for integrating with the search engine. Its responsibility is to run the Solr expression, based on the search profile attributes, and capture the response from the search engine.
A query preprocessor is used to modify the SolrQuery object right before it is sent to the Solr server for processing.
A query postprocessor is used to modify the QueryResponse object that is returned from the Solr server.