Concurrent active connections
- An active database server connection is ready to process SQL requests.
The major advantage of a thread-safe application is that each thread can have one active connection to a database server. Use the CONNECT statement to establish a connection and make it active. Use the SET CONNECTION statement (with no DORMANT clause) to make a dormant connection active.
- A dormant database server connection was established but is not
currently associated with a thread.
When a thread makes an active connection dormant, that connection becomes available to other threads. Conversely, when a thread makes a dormant connection active, that connection becomes unavailable to other threads. Use the SET CONNECTION...DORMANT statement to explicitly put a connection in a dormant state.
The current connection is the active database server connection that is currently sending SQL requests to, and possibly receiving data from, the database server. A single-threaded application has only one current (or active) connection at a time. In a multithreaded application, each thread can have a current connection. Thus a multithreaded application can have multiple active connections simultaneously.
When you switch connections with the SET CONNECTION statement (with no DORMANT clause), SET CONNECTION implicitly puts the current connection in the dormant state. When in a dormant state, a connection is available to other threads. Any thread can access any dormant connection. However, a thread can only have one active connection at a time.
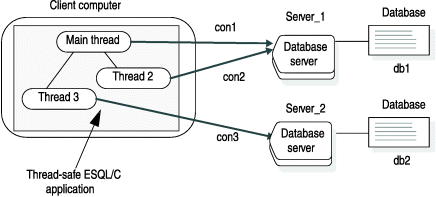
- The main thread (main function) starts connection con1 to database db1 on Server_1.
- The main thread creates Thread 2. Thread 2 establishes connection con2 to database db1 on Server_1.
- The main thread creates Thread 3. Thread 3 establishes connection con3 to database db2 on Server_2.
main()
{
EXEC SQL connect to 'db1@Server_1' as 'con1';
start_threads(); /* start 2 threads */
EXEC SQL select a into :a from t1; /* table t1 resides in db1 */
⋮
}
thread_1()
{
EXEC SQL connect to 'db1@Server_1' as 'con2';
EXEC SQL insert into table t2 values (10); /* table t2 is in db1 */
EXEC SQL disconnect 'con2';
}
thread_2()
{
EXEC SQL connect to 'db2@Server_2' as 'con3';
EXEC SQL insert into table t1 values(10); /* table t1 resides in db2
*/
EXEC SQL disconnect 'con3';
}
You can use the ifx_getcur_conn_name() function to obtain the name of the current connection.