Fetch rows into a cursor
When mi_open_prepared_statement() successfully opens a cursor, the cursor is empty, with the cursor position pointing to the first location of the cursor, and the mi_get_result() function returns a status of MI_NO_MORE_RESULTS to indicate that the cursor does not contain rows.
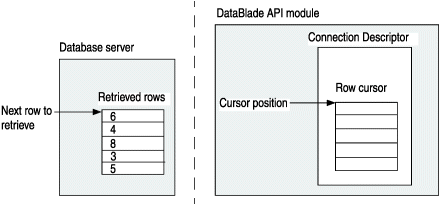
- The statement descriptor for the prepared statement that returns rows
- The location in the rows on the database server at which to begin the fetch
- The number of rows to fetch into the cursor
- The cursor contains the number of rows that the num_rows argument specifies.
- The cursor position points to the first of the fetched rows in the cursor.
- The mi_get_result() function returns a status of MI_ROWS to indicate that the cursor does contain rows.
Cursor-action flag | Description | Type of cursor |
---|---|---|
MI_CURSOR_NEXT | Fetches the next num_rows rows, starting at the current retrieved row on the database server | Sequential Scroll |
MI_CURSOR_PRIOR | Fetches the previous num_rows rows, starting at the current retrieved row | Scroll |
MI_CURSOR_FIRST | Fetches the first num_rows rows | Sequential Scroll |
MI_CURSOR_LAST | Fetches the last num_rows rows | Sequential Scroll |
MI_CURSOR_ABSOLUTE | Moves jump rows into the retrieved rows and fetches num_rows rows | Sequential (as long as the jump argument
does not move the cursor position backward) Scroll |
MI_CURSOR_RELATIVE | Moves jump rows from the current retrieved row and fetch num_rows rows | Sequential (as long as the jump argument
is a positive number) Scroll |
mi_fetch_statement(stmt_desc, MI_CURSOR_NEXT, 0, 0);
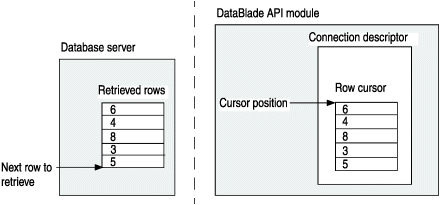
After the rows are in the cursor, your DataBlade® API module can access these rows one at a time with the mi_next_row() function. For more information, see Retrieving query data.
- If you do not need to examine additional rows, exit the mi_next_row() and mi_get_result() loops normally and close the cursor with mi_close_statement().
- If you do need to fetch any rows remaining on the database server
into the cursor, execute the mi_fetch_statement() function
again after the following conditions occur:
- The mi_get_result() function returns MI_DML (for a SELECT statement).
- The number of query rows that mi_next_row() obtains
is less than the number of rows that mi_fetch_statement() fetches
(num_rows) from the database server.
You can obtain the number of query rows with the mi_result_row_count() function.
mi_fetch_statement(stmt_desc, MI_CURSOR_NEXT, 0, 3);
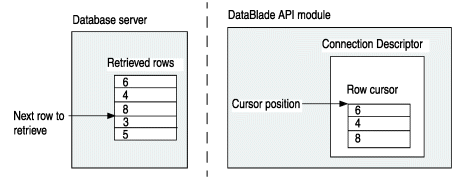
mi_string *cmd =
"select order_num from orders \
where customer_num = ?;";
MI_STATEMENT *stmt;
...
if ( (stmt = mi_prepare(conn, cmd, NULL)) == NULL )
mi_db_error_raise(NULL, MI_EXCEPTION,
"mi_prepare() failed");
values[0] = 104;
types[0] = "integer";
lengths[0] = 0;
nulls[0] = MI_FALSE;
/* Open the read-only cursor to hold the query rows */
if ( mi_open_prepared_statement(stmt, MI_SEND_READ,
MI_TRUE, 1, values, lengths, nulls, types,
"cust_select", retlen, rettypes)
!= MI_OK )
mi_db_error_raise(NULL, MI_EXCEPTION,
"mi_open_prepared_statement() failed");
/* Fetch the retrieved rows into the cursor */
if ( mi_fetch_statement(stmt, MI_CURSOR_NEXT, 0, 3) != MI_OK )
mi_db_error_raise(NULL, MI_EXCEPTION,
"mi_fetch_statement() failed");
if ( mi_get_result(conn) != MI_ROWS )
mi_db_error_raise(NULL, MI_EXCEPTION,
"mi_get_result() failed or found nonquery statement");
/* Retrieve the query rows from the cursor */
if ( !(get_data(conn)) )
mi_db_error_raise(NULL, MI_EXCEPTION,
"get_data() failed");
/* Close the cursor */
if ( mi_close_statement(stmt) == MI_ERROR )
mi_db_error_raise(NULL, MI_EXCEPTION,
"mi_close_statement() failed");
/* Release resources */
if ( mi_drop_prepared_statement(stmt) == MI_ERROR )
mi_db_error_raise(NULL, MI_EXCEPTION,
"mi_drop_prepared_statement() failed");
if ( mi_close(conn) == MI_ERROR )
mi_db_error_raise(NULL, MI_EXCEPTION,
"mi_close() failed");
This code fragment sends its input-parameter value in binary representation. The code fragment is part of a C UDR because it passes the INTEGER input-parameter value by value. For more information, see Assign values to input parameters.