Encrypting data in custom code using EncryptionFactory
EncryptionFactory is a factory class that initializes all of the encryption provider classes that are used at runtime for encrypting and decrypting data. All encryption providers must implement the com.ibm.commerce.foundation.common.util.encryption.EncryptionProvider interface.
The following are the encryption providers that you can use for
encrypting / decrypting data:
- ActiveProvider: This provider is responsible for encrypting and decrypting sensitive data to be stored in the database, for example credit card numbers. The encryption key is the merchant key.
- SessionProvider: This provider is responsible for encrypting and decrypting external facing data such as values in a cookie. The encryption key is the session key.
EncryptionFactory.getInstance().getProvider("name of provider")
To encrypt data that uses an encryption provider, call the encrypt() method.
For example, to encrypt data to be stored in the database, use the ActiveProvider
(merchant
key):com.ibm.commerce.foundation.common.util.encryption.EncryptionFactory.getInstance()
.getProvider(com.ibm.commerce.server.ECConstants.EC_ENCRYPTION_ACTIVEPROVIDER)
.encrypt("myDataToEncrypt");
The following interaction diagram
explains how a component encrypts sensitive data:
- Component first calls the EncryptionFactory to return an instance of the EncryptionProvider given the name of the provider, for example, ActiveProvider.
- Component calls the encrypt method on the provider by passing in the plain text data.
- The provider first calls the WCKeyRegistry to retrieve the key: the new key if it exists, or else the current key with the highest version number. This key is used to encrypt the clear text data. The encrypted data is then returned back to the component.
com.ibm.commerce.foundation.common.util.encryption.EncryptionFactory.getInstance()
.getProvider("com.ibm.commerce.server.ECConstants.EC_ENCRYPTION_ACTIVEPROVIDER")
.decrypt("myEncryptedData");
The following interaction diagram demonstrates how a
component decrypts data: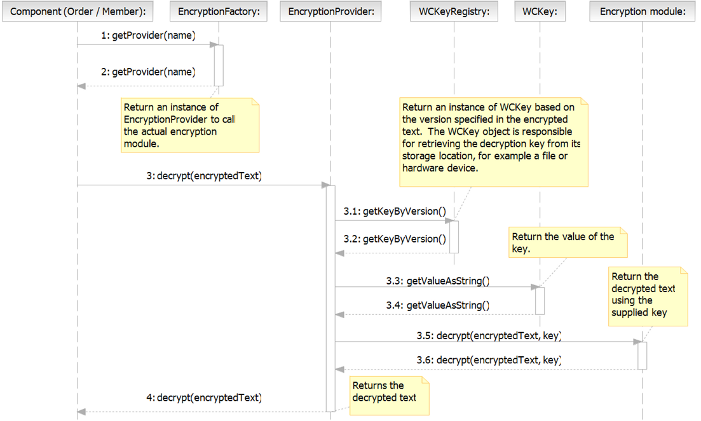
- Component first calls the EncryptionFactory to return an instance of the EncryptionProvider given a name.
- Component calls the decrypt method on the provider by passing in the encrypted data.
- The provider determines the version of the key that was used to encrypt the data by parsing the plain text suffix. Then, it calls the WCKeyRegistry to retrieve the key with the same version. Finally, it calls the encryption module to decrypt the data that uses the key.
Note: If you are using EncryptionFactory in a command line
envrionment, and are using the Key Locator Framework, then the following code is needed
before you call the EncryptionFactory
API.
System.setProperty("newKeyNeeded", "true");
com.ibm.commerce.security.keys.WCKeyRegistry keyRegistry = com.ibm.commerce.security.keys.WCKeyRegistry.getInstance();
if (!keyRegistry.isInitialized()) {
keyRegistry.initFromXMLFile( pathToCustomKeysFile );
}